안녕하세요!
오늘은 Spring boot와 react Axios 통신 중 CORS 오류 문제를 해결하는 방법에 대해 소개해 드리겠습니다.
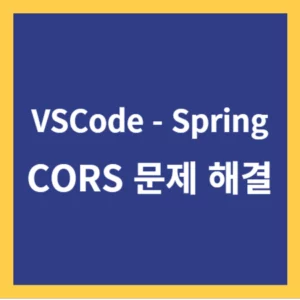
CORS
CORS는 Cross-Origin Resource Sharing의 약자로 웹 애플리케이션에서 다른 도메인, 포트 또는 프로토콜의 리소스에 접근할 수 있는 메커니즘을 합니다,
다른 도메인 간 요청에 대한 보안을 강화하기 위해 다른 출처에 리소스를 요청할 때 해당 리소스가 교차 출처 리소스 공유 정책에 따라 접근 가능한지 확인하고 허용하는 방법입니다.
그래서 다른 API나 포트가 다른 프로젝트를 진행할 때 CORS 문제를 해결하여 리소스를 요청할 수 있습니다.
Axios
Axios는 브라우저나 Node.js에서 주로 사용되는 비동기 통신 방식으로 선호도가 높은 라이브러리 중 하나인 기능이에요.
이번 포스팅에서는 Axios 기능을 사용하여 문제를 해결해 보겠습니다.
프로젝트 생성
일반적으로 react 애플리케이션을 빌드하고 spring boot에 포함시키면 CORS 문제는 발생하지 않지만
build를 하기 전에 개발할 때 따로 개발하는 경우가 있어서 오류가 발생하게 됩니다.
react에서는 VSCode, Spring boot는 spring tool로 비동기 통신을 해보겠습니다.
Spring boot 프로젝트 생성하는 방법은 이곳에 있으니 참고해 주시길 바랍니다!
Spring boot 설치부터 프로젝트 만들기 / 실행까지!
axios 라이브러리 설치
npx create-react-app 프로젝트이름
npm install axios
먼저 VSCode에 react를 설치한 뒤 axios를 설치합니다.
다음 spring과 통신하는 코드를 작성합니다.
// react 코드
import './App.css';
import React, { useEffect, useState } from 'react';
import axios from "axios"
function App() {
// 통신 받은 결과 값 변수
const [val, setValue] = useState(null);
// 화면이 실행 될때 한 번만 실행하기
useEffect(()=>{
// axios 통신
axios("/hello")
.then(res => {
console.log(res.data)
setValue(res.data)
})
},[])
return (
<div className="App">
{val}
</div>
);
}
export default App;
package com.hyehwi.app.controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class HelloController {
@RequestMapping("/hello")
public String hello() {
return "성공~!";
}
}
통신하는 코드를 작성했다면 문제를 해결해 보겠습니다.
react에서 해결하는 방법(setupProxy.js 생성)
const { createProxyMiddleware } = require('http-proxy-middleware');
module.exports = function(app) {
app.use(
'/', // 프론트엔드에서 요청하는 백엔드 API의 경로
createProxyMiddleware({
target: 'http://localhost:8089', // 백엔드 서버의 주소
changeOrigin: true, // 요청 헤더의 호스트를 대상 서버로 변경
})
);
};
첫 번째 방법은 react의 proxy를 바꿔서 문제를 해결하는 방법입니다.
react 파일 경로의 src 폴더에 setupProxy.js 파일을 만들고 위의 코드를 작성합니다.
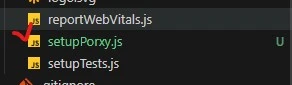
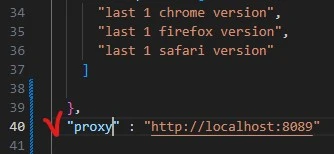
그다음 package.json 파일로 이동하고 맨 마지막에 Spring boot의 포트 번호에 맞게 작성합니다.
Spring boot에서 CORS해결
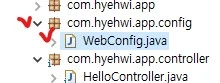
다음은 Spring boot에서 react의 리소스 요청을 허용하는 방식입니다.
스프링 src/main/java 폴더에 config 패키지를 새로 만들고 java 파일을 만들고 다음과 같은 코드를 작성합니다.
package com.hyehwi.app.config;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebConfig implements WebMvcConfigurer{
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**")
.allowedOrigins("http://localhost:3000")
.allowedMethods("GET", "POST", "PUT","DELETE")
.allowedHeaders("*")
.maxAge(3600);
}
}
VSCode의 react 포트 번호 3000번에 대한 메소드들을 허용하는 코드입니다.
이 코드가 완성되었다면 마지막으로 react의 axios 코드를 spring boot로 보내는 코드로 변경합니다.
axios("http://localhost:8089/hello")
.then(res => {
console.log(res.data)
setValue(res.data)
})
결과 확인
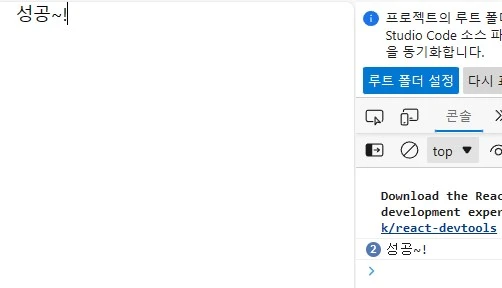
결과를 확인한 결과 spring boot에서 받아온 데이터를 화면에 띄우는 것까지 성공했습니다!
여기까지 VSCode와 Spring boot의 CORS 문제 해결이었습니다.
다음에도 좋은 정보로 찾아뵙겠습니다.