안녕하세요!
오늘은 비동기 통신 처리 방식 함수 중 jquery에서 Promise, react에서 async, await에 대해 소개해 드려요!
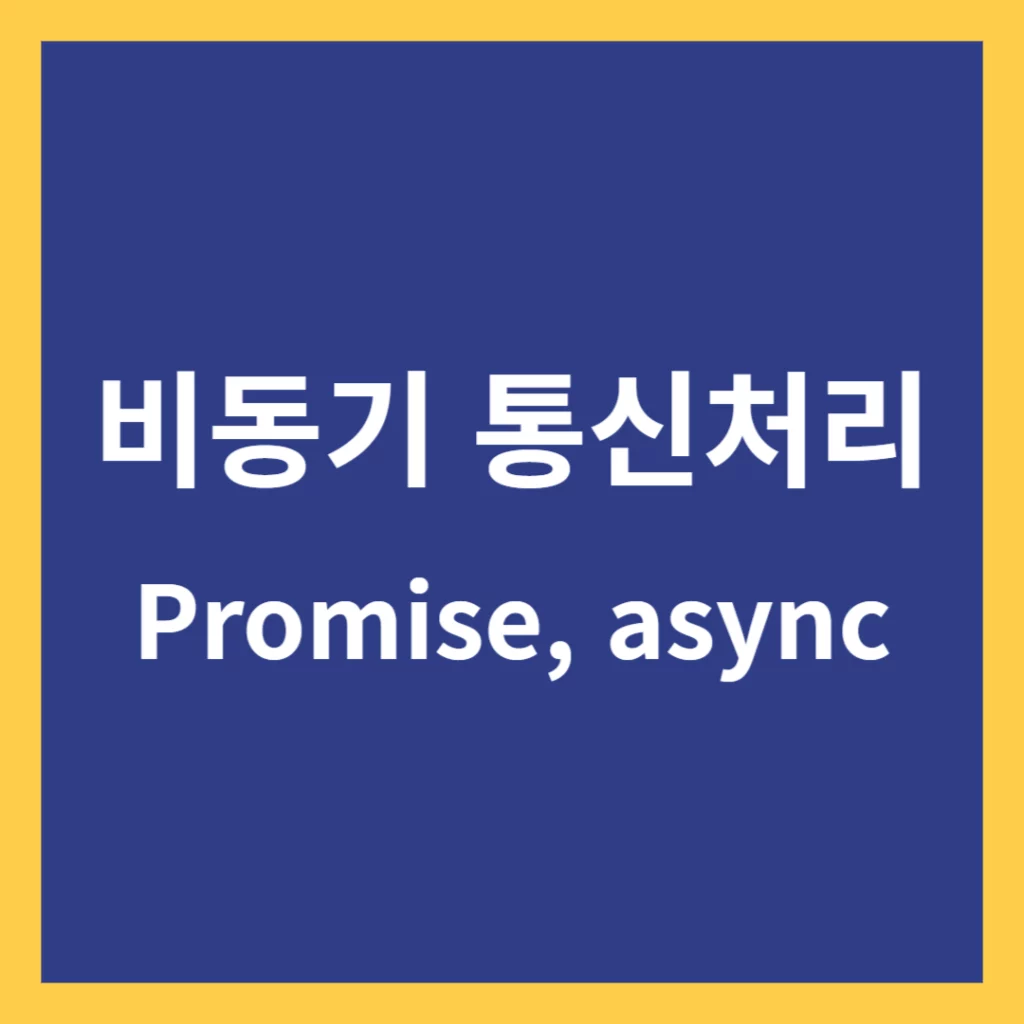
비동기 통신 방식이란?
웹 애플리케이션을 개발하다 보면 데이터를 가져오는 상황이 많이 발생하게 됩니다.
여기서 페이지를 새로고침 하지 않고 필요한 부분에 데이터를 가져와서 화면을 전환하는 기술을 비동기 통신이라고 합니다.
이런 기술들을 쉽게 접하실 수 있는데요.
인스타그램 페이지도 비동기 통신 방식 사용한 기능이 있어요!
비동기 통신 종류
fetch('/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
axios.get('/data')
.then(response => console.log(response.data))
.catch(error => console.error(error));
$.ajax({
url : "",
type : "",
data : "",
dataType : "json",
success : function(res){
// 통신 성공시 실행
},
error : function(error){
// 통신 실패시 실행
}
})
비동기 통신의 종류는 다양한데 주로 Fetch, Axios, Ajax가 있어요.
여기에서 만약 비동기 통신으로 받아온 데이터 사용해야 하는데 데이터가 받지 못하고 코드가 실행됐다면 어떻게 해야 할까요?
바로 Promise와 asncy, await가 있습니다!
Promise 함수
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Promise and AJAX Example</title>
<!-- jQuery 라이브러리 추가 -->
<script src="https://code.jquery.com/jquery-3.6.4.min.js"></script>
</head>
<body>
<button id="loadDataBtn">Load Data</button>
<div id="result"></div>
<script>
// AJAX 요청을 Promise로 감싸는 함수
function fetchData() {
return new Promise((resolve, reject) => {
$.ajax({
url: 'https://url',
type: 'GET',
dataType: 'json',
success: function(response) {
resolve(response);
},
error: function(xhr, status, error) {
reject(error);
}
});
});
}
fetchData().then(response => {
// 성공적으로 데이터를 받아왔을 때의 처리
console.log(response);
}).catch(error => {
// 오류 발생 시의 처리
console.error('Error:', error);
});
이 코드에서는 fetchData 함수를 사용하여 AJAX 요청을 보내고 Promise 객체를 반환합니다.
여기서 요청이 성공하면 resolve를 호출하고 실패하면 reject를 호출합니다.
다음 fetchData().then을 사용하여 AJAX에서 받아온 데이터를 이용하여 함수를 사용할 수 있습니다.
첫 번째 비동기 데이터를 받고 두 번째 비동기 통신도 할 수 있습니다!
async, await
import React, { useState, useEffect } from 'react';
import axios from 'axios';
const App = () => {
const [data, setData] = useState(null);
const [error, setError] = useState(null);
const fetchData = async () => {
try {
const response = await axios.get('https://url');
setData(response.data);
} catch (error) {
setError(error.message);
}
};
useEffect(() => {
fetchData();
}, []); // 빈 배열은 컴포넌트가 마운트될 때 한 번만 실행
return (
<div>
<h1>React Async/Await with Axios</h1>
{error && <p>Error: {error}</p>}
{data && (
<div>
<h2>{data.title}</h2>
<p>{data.body}</p>
</div>
)}
</div>
);
};
export default App;
다음은 react에서 Axios로 async, await 방식입니다.
axios 함수에 async와 await를 사용하여 데이터를 받을 때까지 기다린 뒤 성공하면 setData를 호출하고 실패하면 setError를 호출합니다.
여기까지 비동기 처리 방식에 대해 소개해 드렸는데요.
다음에도 좋은 정보로 찾아오겠습니다.